6. テキストを表示する
6.1 デバッグ表示機能 (1)
Print << U"...";
で文字列 ... をデバッグ出力できます
- ダブルクォーテーションの直前に大文字の U が付くことに注意します(文字エンコーディングを UTF-32 にするための文法)
- デバッグ出力したものは画面に残り続けます
- 改行文字が無くても自動的に改行されます
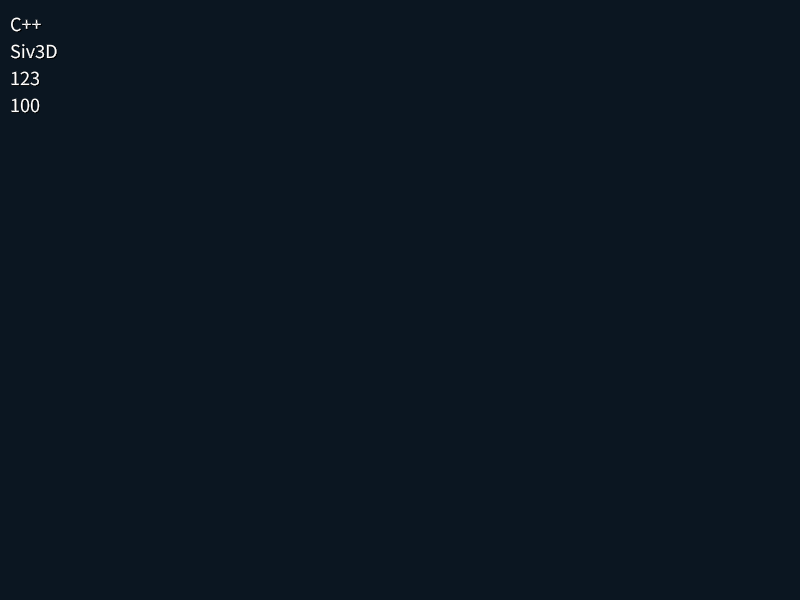
# include <Siv3D.hpp>
void Main()
{
Print << U"C++";
Print << U"Siv3D";
Print << 123;
int32 a = 100;
Print << a;
while (System::Update())
{
}
}
6.2 デバッグ表示機能 (2)
- 画面に収まらなくなったデバッグ表示は古いものから消去されます
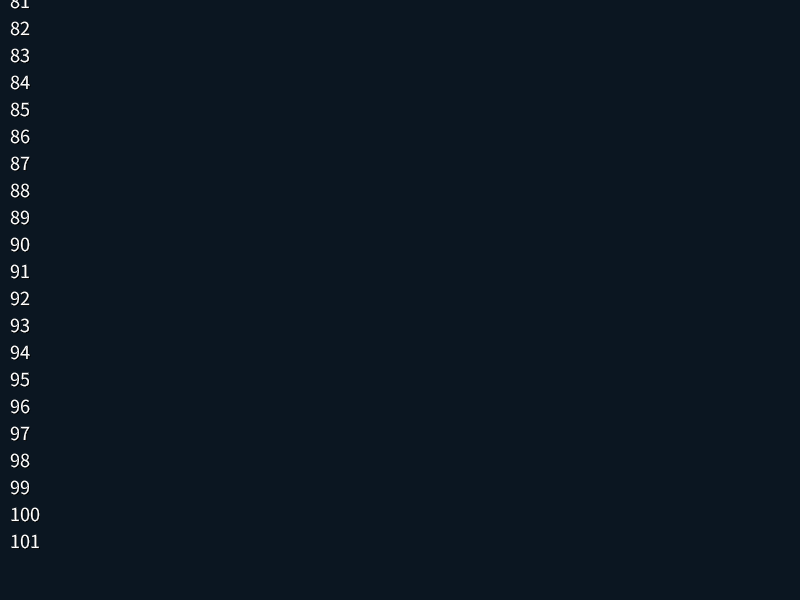
# include <Siv3D.hpp>
void Main()
{
int32 count = 0;
while (System::Update())
{
Print << count;
++count;
}
}
6.3 デバッグ表示機能 (3)
ClearPrint()
を呼ぶとデバッグ表示をすべて消去します
- メインループ内の先頭で
ClearPrint()
することで、現在のフレームの情報だけを表示できます
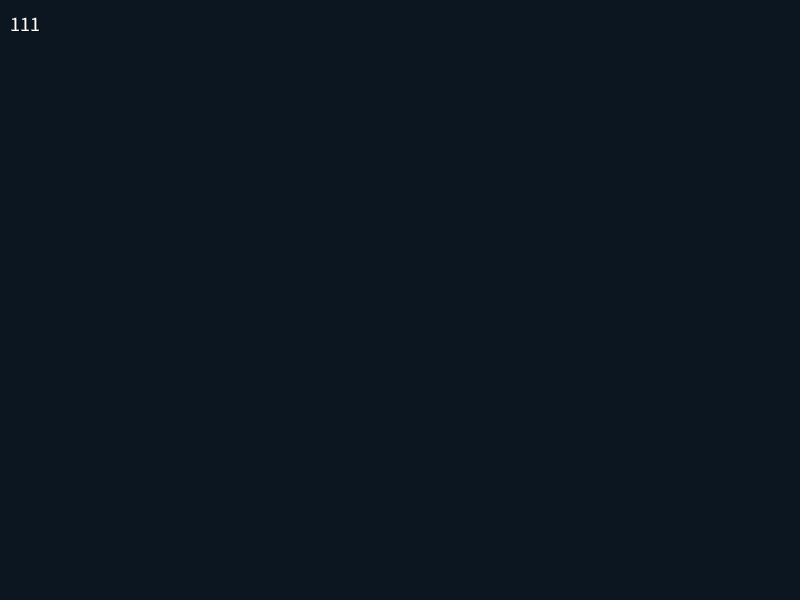
# include <Siv3D.hpp>
void Main()
{
int32 count = 0;
while (System::Update())
{
ClearPrint();
Print << count;
++count;
}
}
6.4 数値を文字列に変換する (1)
U"{}"_fmt(x)
と書くと、{}
には x を文字列にしたものが入ります
U"{} 月 {} 日"_fmt(12, 31)
は U"12 月 31 日"
という文字列になります
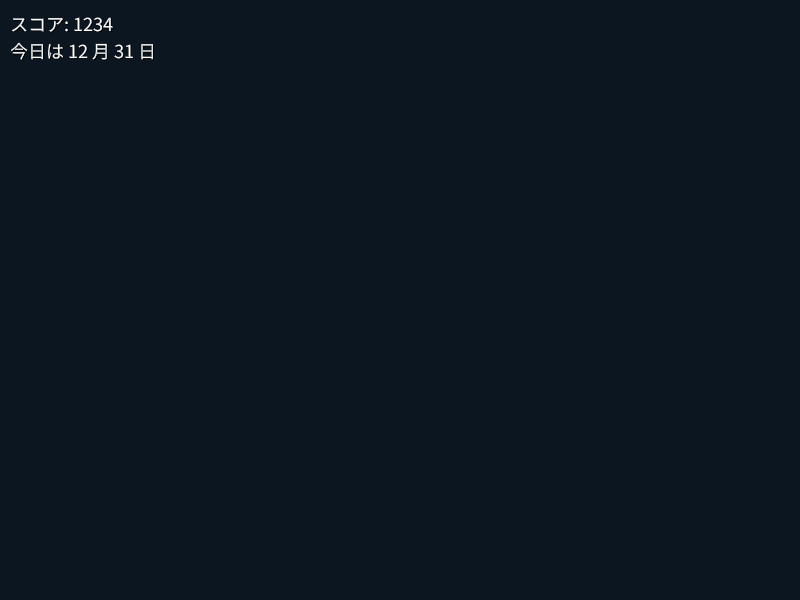
# include <Siv3D.hpp>
void Main()
{
int32 score = 1234;
Print << U"スコア: {}"_fmt(score);
int32 month = 12;
int32 day = 31;
Print << U"今日は {} 月 {} 日"_fmt(month, day);
while (System::Update())
{
}
}
6.5 数値を文字列に変換する (2)
double
型の値 x
を、小数点以下の桁数を指定して変換する場合、U"{:.2f}"_fmt(x)
のように書きます(この場合小数点以下 2 桁)
- 小数点以下を表示しない場合は
U"{:.0f}"_fmt(x)
とします
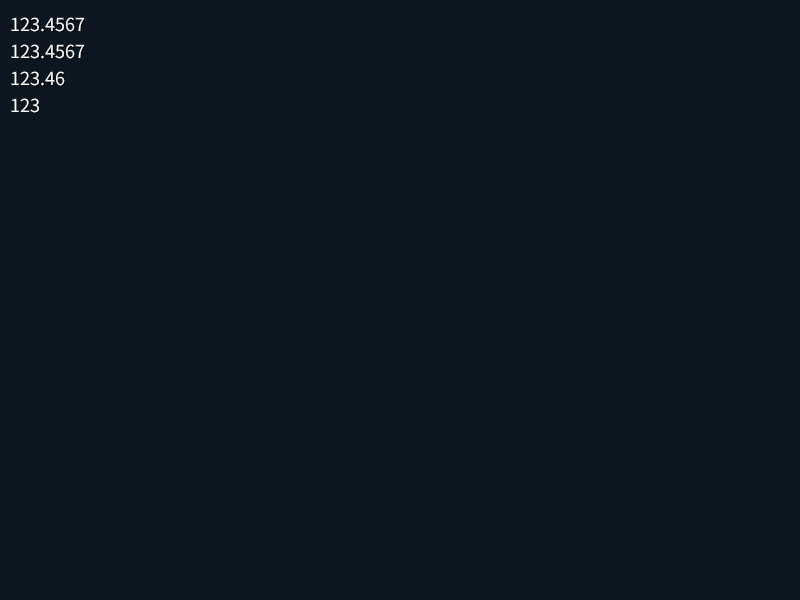
# include <Siv3D.hpp>
void Main()
{
double x = 123.4567;
Print << x;
Print << U"{}"_fmt(x);
Print << U"{:.2f}"_fmt(x);
Print << U"{:.0f}"_fmt(x);
while (System::Update())
{
}
}
6.6 テキストを表示する
- メインループの前に
Font font{ FontMethod::MSDF, 48 };
でフォントを作成します
- フォントの作成はコストがかかるため、メインループの前で作成します
- 作成したフォントを使って、
font(テキスト).draw(サイズ, X 座標, Y 座標, 色);
でテキストを表示できます
font(テキスト)
のテキストの部分は、数も使えます
-.draw(サイズ, X 座標, Y 座標);
のように色を省略すると、白色になります
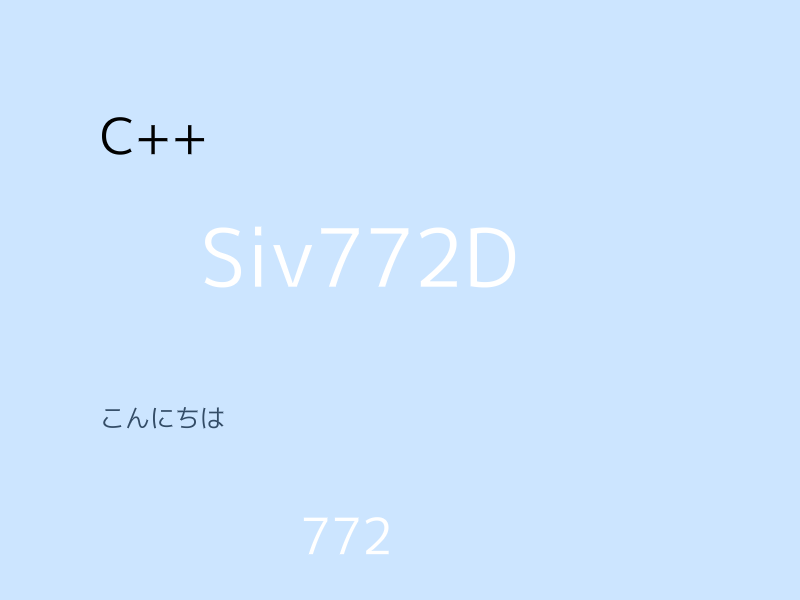
# include <Siv3D.hpp>
void Main()
{
Scene::SetBackground(ColorF{ 0.8, 0.9, 1.0 });
Font font{ FontMethod::MSDF, 48 };
int32 count = 0;
while (System::Update())
{
font(U"C++").draw(50, 100, 100, Palette::Black);
font(U"Siv{}D"_fmt(count)).draw(80, 200, 200);
font(U"こんにちは").draw(25, 100, 400, ColorF{ 0.2, 0.3, 0.4 });
font(count).draw(50, 300, 500);
++count;
}
}
6.7 太文字のテキストを表示する
- 太文字のフォントは
Font font{ FontMethod::MSDF, 48, Typeface::Bold };
で作成できます
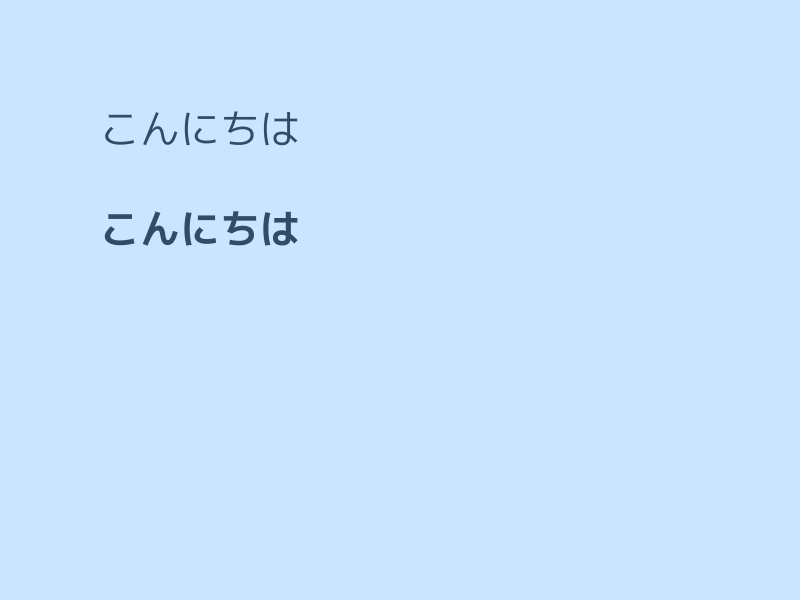
# include <Siv3D.hpp>
void Main()
{
Scene::SetBackground(ColorF{ 0.8, 0.9, 1.0 });
Font normalFont{ FontMethod::MSDF, 48 };
// 太文字のフォント
Font boldFont{ FontMethod::MSDF, 48, Typeface::Bold };
while (System::Update())
{
normalFont(U"こんにちは").draw(40, 100, 100, ColorF{ 0.2, 0.3, 0.4 });
boldFont(U"こんにちは").draw(40, 100, 200, ColorF{ 0.2, 0.3, 0.4 });
}
}
6.8 テキストの基準位置を変更する
- 中心の座標を指定してテキストを表示するには
.drawAt(サイズ, X 座標, Y 座標, 色);
を呼びます
- 右端の中心の座標を指定してテキストを表示するには
.draw(サイズ, Arg::rightCenter(X 座標, 座標), 色);
を呼びます
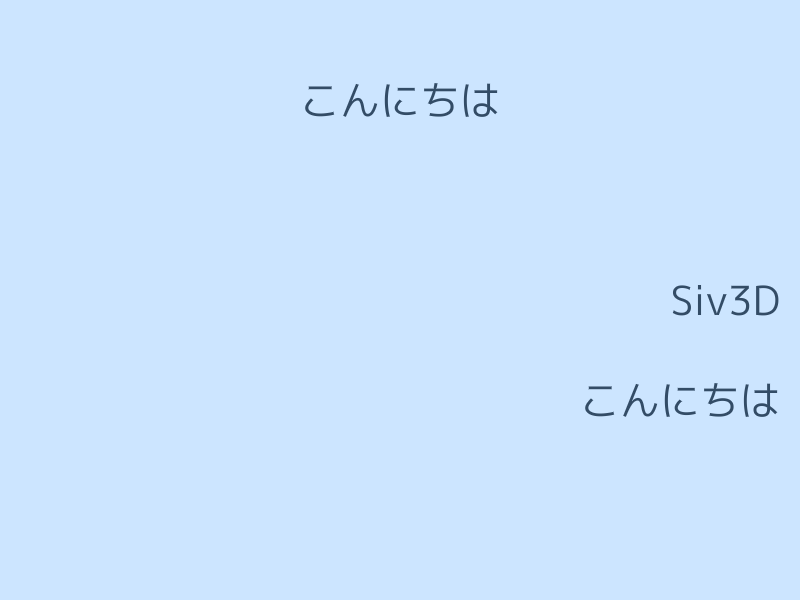
# include <Siv3D.hpp>
void Main()
{
Scene::SetBackground(ColorF{ 0.8, 0.9, 1.0 });
Font font{ FontMethod::MSDF, 48 };
while (System::Update())
{
font(U"こんにちは").drawAt(40, 400, 100, ColorF{ 0.2, 0.3, 0.4 });
font(U"Siv3D").draw(40, Arg::rightCenter(780, 300), ColorF{ 0.2, 0.3, 0.4 });
font(U"こんにちは").draw(40, Arg::rightCenter(780, 400), ColorF{ 0.2, 0.3, 0.4 });
}
}