8. キーボード入力を扱う
8.1 キーボードが押されたか調べる
キー名.down()
で、あるキーが押されたかを調べられます
キー名
- A, B, C, ... は
KeyA
, KeyB
, KeyC
, ...
- 1, 2, 3, ... は
Key1
, Key2
, Key3
, ...
- F1, F2, F3, ... は
KeyF1
, KeyF2
, KeyF3
, ...
- ↑, ↓, ←, → は
KeyUp
, KeyDown
, KeyLeft
, KeyRight
- スペースキーは
KeySpace
- エンターキーは
KeyEnter
- バックスペースキーは
KeyBackspace
- Tab キーは
KeyTab
- Esc キーは
KeyEscape
- PageUp, PageDown は
KeyPageUp
, KeyPageDown
- Delete キーは
KeyDelete
- Numpad の 0, 1, 2, ... は
KeyNum0
, KeyNum1
, KeyNum2
, ...
- シフトキーは
KeyShift
- 左シフトキー、右シフトキーは
KeyLShift
, KeyRShift
- コントロールキーは
KeyControl
- (macOS) コマンドキーは
KeyCommand
- 「,」「.」「/」キーは
KeyComma
, KeyPeriod
, KeySlash
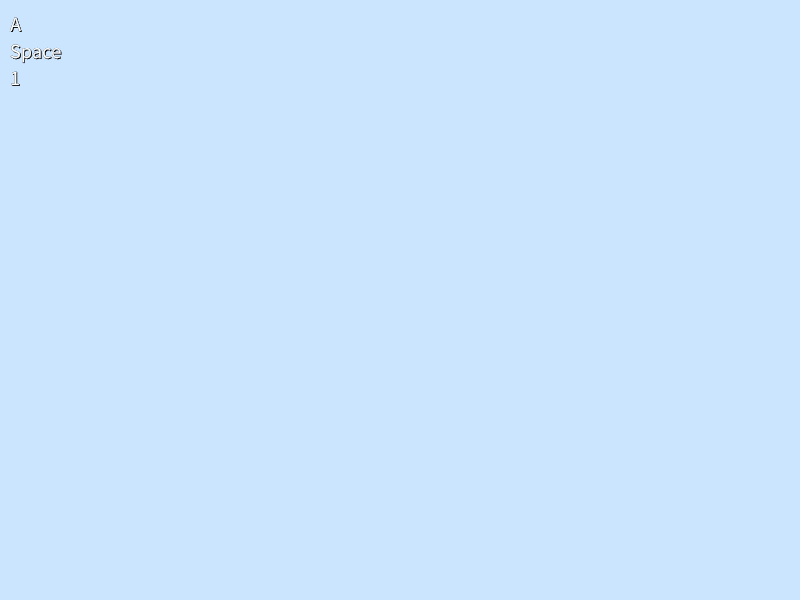
# include <Siv3D.hpp>
void Main()
{
Scene::SetBackground(ColorF{ 0.8, 0.9, 1.0 });
while (System::Update())
{
// A キーが押されたら
if (KeyA.down())
{
Print << U"A";
}
// スペースキーが押されたら
if (KeySpace.down())
{
Print << U"Space";
}
// 1 キーが押されたら
if (Key1.down())
{
Print << U"1";
}
}
}
8.2 キーボードが押されているか調べる
キー名.pressed()
で、あるキーが押されているかを調べられます
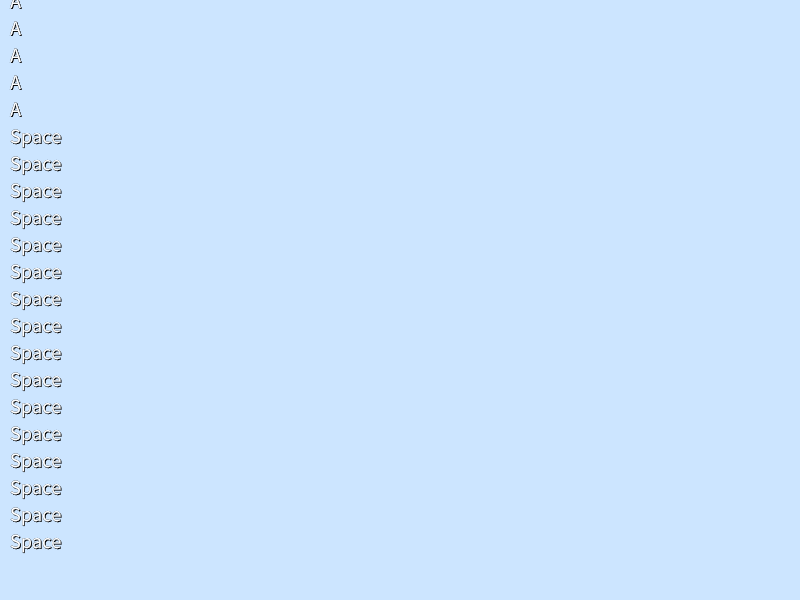
# include <Siv3D.hpp>
void Main()
{
Scene::SetBackground(ColorF{ 0.8, 0.9, 1.0 });
while (System::Update())
{
// A キーが押されていたら
if (KeyA.pressed())
{
Print << U"A";
}
// スペースキーが押されていたら
if (KeySpace.pressed())
{
Print << U"Space";
}
// 1 キーが押されていたら
if (Key1.pressed())
{
Print << U"1";
}
}
}
8.3 キーボードで左右に移動する
- 矢印キーを使って絵文字を左右に移動させるには次のようにします
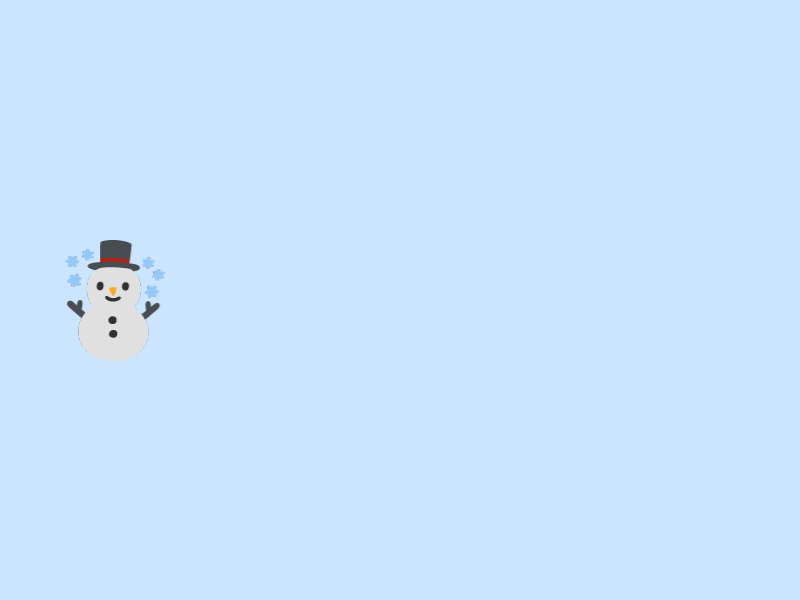
# include <Siv3D.hpp>
void Main()
{
Scene::SetBackground(ColorF{ 0.8, 0.9, 1.0 });
Texture emoji{ U"☃️"_emoji };
// 移動の速さ(ピクセル / 秒)
double speed = 200.0;
double x = 400.0;
while (System::Update())
{
double deltaTime = Scene::DeltaTime();
// ← キーが押されていたら
if (KeyLeft.pressed())
{
x -= (speed * deltaTime);
}
// → キーが押されていたら
if (KeyRight.pressed())
{
x += (speed * deltaTime);
}
emoji.drawAt(x, 300);
}
}
上下左右に移動するサンプル(開く前に考えてみよう)
# include <Siv3D.hpp>
void Main()
{
Scene::SetBackground(ColorF{ 0.8, 0.9, 1.0 });
Texture emoji{ U"☃️"_emoji };
// 移動の速さ(ピクセル / 秒)
double speed = 200.0;
double x = 400.0;
double y = 300.0;
while (System::Update())
{
double deltaTime = Scene::DeltaTime();
// ← キーが押されていたら
if (KeyLeft.pressed())
{
x -= (speed * deltaTime);
}
// → キーが押されていたら
if (KeyRight.pressed())
{
x += (speed * deltaTime);
}
// ↑ キーが押されていたら
if (KeyUp.pressed())
{
y -= (speed * deltaTime);
}
// ↓ キーが押されていたら
if (KeyDown.pressed())
{
y += (speed * deltaTime);
}
emoji.drawAt(x, 300);
}
}